برمجة لعبة X | O
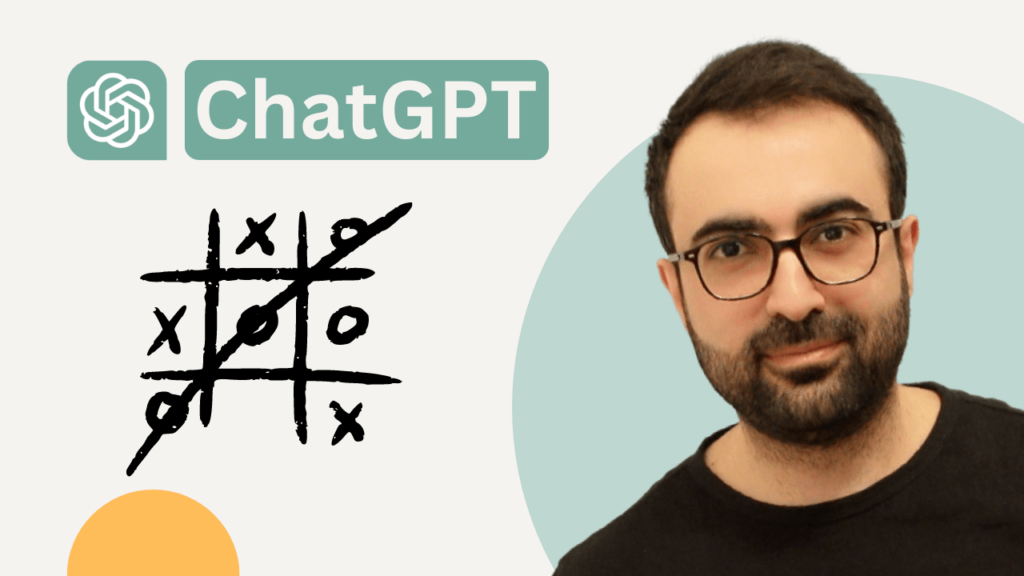
الأمر الموجه لـ ChatGPT
إنني أتطلع إلى إنشاء لعبة Tic-Tac-Toe بسيطة يمكن تشغيلها على متصفح الويب. يجب أن يتم استخدام HTML للهيكل، وCSS لتحسين المظهر، وJavaScript لوظائف اللعبة. تحتاج اللعبة إلى دعم لاعبين اثنين على نفس الجهاز. يجب أن يكون كل لاعب قادراً على النقر على خلية فارغة في شبكة 3×3 لوضع علامة على الرمز الخاص به (X أو O). يجب أن تتحقق اللعبة من الفوز أو التعادل بعد كل نقلة، وتعرض رسالة تعلن الفائز أو التعادل عندما يكون ذلك مناسباً. بالإضافة إلى ذلك، يجب أن يكون هناك أزرار لإعادة تشغيل اللعبة وإعادة ضبط النتيجة. يجب أن يكون التصميم نظيفاً وسريع الاستجابة ليناسب الأجهزة المختلفة. هل يمكنك توفير الكود لتحقيق ذلك؟
HTML
يتضمن هذا الجزء البنية الأساسية للوحة اللعبة، وأزرار إعادة تشغيل اللعبة، وإعادة ضبط النتيجة، بالإضافة إلى العناصر الأساسية لرسالة حالة اللعبة.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Tic-Tac-Toe Game</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="game">
<div id="scoreboard">
<div id="scoreX">Player X: 0</div>
<div id="scoreO">Player O: 0</div>
</div>
<div id="gameStatus" class="status">Player X's turn</div>
<div class="board" id="board">
<div class="cell" data-cell-index="0"></div>
<div class="cell" data-cell-index="1"></div>
<div class="cell" data-cell-index="2"></div>
<div class="cell" data-cell-index="3"></div>
<div class="cell" data-cell-index="4"></div>
<div class="cell" data-cell-index="5"></div>
<div class="cell" data-cell-index="6"></div>
<div class="cell" data-cell-index="7"></div>
<div class="cell" data-cell-index="8"></div>
</div>
<button id="restartButton">Restart Game</button>
<button id="resetScore">Reset Score</button>
</div>
<script src="script.js"></script>
</body>
</html>
CSS
يضيف هذا الجزء تصميماً أساسياً إلى لوحة اللعبة والخلايا، مما يجعل اللعبة سريعة الاستجابة ونظيفة بصرياً
body, html {
height: 100%;
margin: 0;
display: flex;
align-items: center;
justify-content: center;
font-family: Arial, sans-serif;
}
.game {
display: flex;
flex-direction: column;
align-items: center;
}
.scoreboard {
margin-bottom: 10px;
font-size: 16px;
}
.board {
display: grid;
grid-template-columns: repeat(3, 100px);
grid-gap: 10px;
margin-bottom: 20px;
}
.cell {
width: 100px;
height: 100px;
background-color: #ddd;
display: flex;
align-items: center;
justify-content: center;
font-size: 40px;
cursor: pointer;
}
.status {
margin-bottom: 20px;
}
Javascript
يدير هذا النصي منطق اللعبة، بما في ذلك أدوار اللاعبين والفوز وإعادة تشغيل اللعبة.
const board = document.getElementById('board');
const cells = document.querySelectorAll('.cell');
const gameStatus = document.getElementById('gameStatus');
const restartButton = document.getElementById('restartButton');
const resetScoreButton = document.getElementById('resetScore');
const scoreX = document.getElementById('scoreX');
const scoreO = document.getElementById('scoreO');
let currentPlayer = 'X';
let gameActive = true;
let gameState = ['', '', '', '', '', '', '', '', ''];
let score = { 'X': 0, 'O': 0 };
const winningConditions = [
[0, 1, 2],
[3, 4, 5],
[6, 7, 8],
[0, 3, 6],
[1, 4, 7],
[2, 5, 8],
[0, 4, 8],
[2, 4, 6]
];
function handleCellPlayed(clickedCell, clickedCellIndex) {
gameState[clickedCellIndex] = currentPlayer;
clickedCell.innerText = currentPlayer;
}
function handlePlayerChange() {
currentPlayer = currentPlayer === 'X' ? 'O' : 'X';
gameStatus.innerText = `Player ${currentPlayer}'s turn`;
}
function updateScores() {
scoreX.innerText = `Player X: ${score['X']}`;
scoreO.innerText = `Player O: ${score['O']}`;
}
function handleResultValidation() {
let roundWon = false;
for (let i = 0; i <= 7; i++) {
const winCondition = winningConditions[i];
let a = gameState[winCondition[0]];
let b = gameState[winCondition[1]];
let c = gameState[winCondition[2]];
if ([a, b, c].includes('')) {
continue;
}
if (a === b && b === c) {
roundWon = true;
break;
}
}
if (roundWon) {
gameStatus.innerText = `Player ${currentPlayer} wins!`;
score[currentPlayer]++;
updateScores();
gameActive = false;
return;
}
let roundTie = !gameState.includes('');
if (roundTie) {
gameStatus.innerText = 'Game is a tie!';
gameActive = false;
return;
}
handlePlayerChange();
}
function handleCellClick(event) {
const clickedCell = event.target;
const clickedCellIndex = parseInt(clickedCell.getAttribute('data-cell-index'));
if (gameState[clickedCellIndex] !== '' || !gameActive) {
return;
}
handleCellPlayed(clickedCell, clickedCellIndex);
handleResultValidation();
}
function handleRestartGame() {
gameActive = true;
currentPlayer = 'X';
gameState = ['', '', '', '', '', '', '', '', ''];
gameStatus.innerText = `Player X's turn`;
cells.forEach(cell => cell.innerText = '');
}
function handleResetScore() {
score = { 'X': 0, 'O': 0 };
updateScores();
handleRestartGame();
}
board.addEventListener('click', handleCellClick);
restartButton.addEventListener('click', handleRestartGame);
resetScoreButton.addEventListener('click', handleResetScore);
الأمر باللغة الإنجليزية لاستجابة أفضل
I’m looking to build a simple Tic-Tac-Toe game that can be played on the web. It should use HTML for the structure, CSS for basic styling, and JavaScript for the game mechanics. The game needs to support two players on the same device. Each player should be able to click on an empty cell in a 3×3 grid to mark their symbol (X or O). The game should check for a win or a tie after every move, and display a message declaring the winner or a tie when appropriate. Additionally, there should be buttons to restart the game and to reset the score. The design should be clean and responsive to fit on various devices. Can you provide the code to achieve this?
Responses