برمجة لعبة فرقعة البالونات
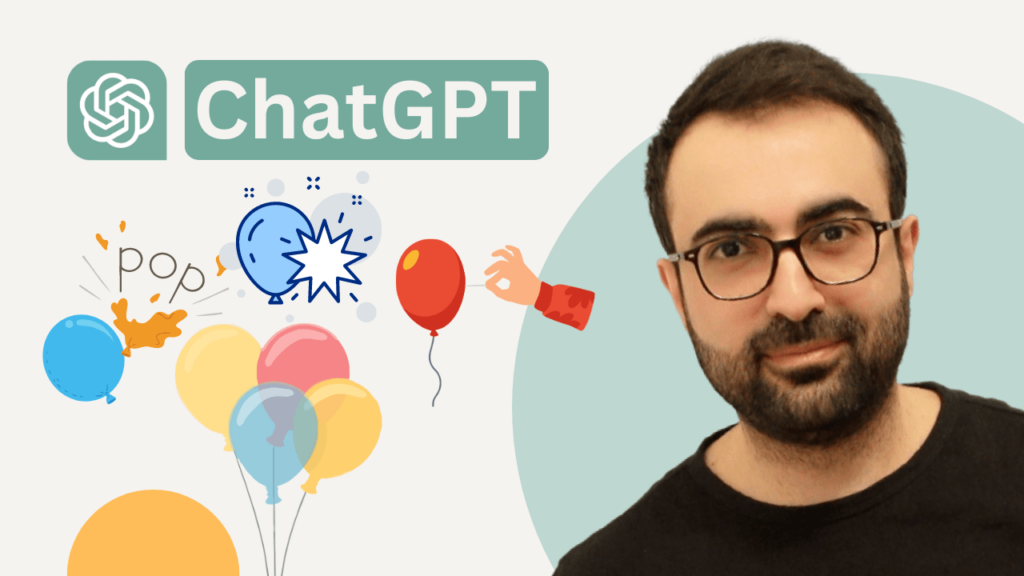
الأمر الموجه لـ ChatGPT
أحتاج إلى تطوير لعبة “Balloon Pop” المبنية باستخدام HTML وCSS وJavaScript. تتضمن اللعبة بالونات بأحجام مختلفة تطفو لأعلى بسرعات مختلفة، ويكسب اللاعبون نقاطاً من خلال النقر عليها. يجب أن تنتهي اللعبة إذا طفت 20 بالونة دون أن يتم النقر عليها، ويجب أن تظهر رسالة تعرض العدد الإجمالي للبالونات التي تم النقر عليها بنجاح. يجب أن يكون هناك أيضاً زر “إعادة تشغيل اللعبة” الذي يسمح للاعبين بالبدء من جديد، وإعادة ضبط اللعبة إلى حالتها الأولية. يجب أن يكون هذا الزر سهل الوصول إليه ومرئياً بوضوح. بالإضافة إلى ذلك، يرجى التأكد من استمرار استجابة اللعبة على كل من أجهزة سطح المكتب والأجهزة المحمولة. هل يمكنك توفير الكود الذي يتضمن آليات اللعبة هذه، أي كود HTML وCSS وJavaScript اللازمة لتنفيذها؟
HTML (Structure)
هذا هو الهيكل الأساسي للعبة. سنقوم بتضمين مناطق لرسائل عرض اللعبة وحاوية البالون وزر إعادة التشغيل.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Balloon Pop Game</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="game-container">
<div class="score-board">
<p>Balloons Popped: <span id="score">0</span></p>
<p>Balloons Missed: <span id="missed">0</span></p>
<button id="restartButton">Restart Game</button>
</div>
<div id="balloon-container"></div>
</div>
<script src="game.js"></script>
</body>
</html>
CSS (Styling)
سوف نتأكد من أن اللعبة جذابة بصرياً وتعمل على كل من الشاشات، سطح المكتب والهاتف المحمول.
/* styles.css */
body, html {
width: 100%;
height: 100%;
margin: 0;
padding: 0;
display: flex;
justify-content: center;
align-items: center;
background-color: #f0f0f0;
}
.game-container {
position: relative;
width: 90%;
max-width: 600px;
height: 80vh;
border: 3px solid #333;
background-color: #fff;
overflow: hidden;
}
.score-board {
text-align: center;
padding: 10px;
background-color: #eee;
}
#balloon-container {
width: 100%;
height: 100%;
position: relative;
overflow: hidden;
}
.balloon {
position: absolute;
width: 50px;
height: 100px;
background-color: red;
border-radius: 50%;
cursor: pointer;
user-select: none;
}
#restartButton {
margin-top: 10px;
padding: 10px 20px;
font-size: 1rem;
cursor: pointer;
}
@media (max-width: 600px) {
.game-container {
width: 100%;
height: 90vh;
}
}
JavaScript (Functionality)
الآن، دعونا نتعامل مع المنطق الرياضي للعبة. يقوم كود JavaScript هذا بإنشاء البالونات وتحريكها، وتتبع النتيجة والبالونات المفقودة، ويسمح بإعادة ضبط اللعبة.
// game.js
document.addEventListener('DOMContentLoaded', function() {
const balloonContainer = document.getElementById('balloon-container');
const scoreDisplay = document.getElementById('score');
const missedDisplay = document.getElementById('missed');
const restartButton = document.getElementById('restartButton');
let score = 0;
let missed = 0;
function createBalloon() {
const balloon = document.createElement('div');
balloon.classList.add('balloon');
var size = Math.random() * 40 + 30; // Balloon size between 30px and 70px
balloon.style.width = `${size}px`;
balloon.style.height = `${size}px`;
balloon.style.left = `${Math.random() * (balloonContainer.clientWidth - size)}px`;
balloon.style.backgroundColor = `hsl(${Math.random() * 360}, 100%, 70%)`;
balloonContainer.appendChild(balloon);
balloon.addEventListener('click', function() {
score++;
scoreDisplay.textContent = score;
balloon.remove();
});
// Move the balloon
let balloonInterval = setInterval(function() {
balloon.style.top = (balloon.offsetTop - 1) + 'px';
if (balloon.offsetTop + balloon.clientHeight < 0) {
missed++;
missedDisplay.textContent = missed;
balloon.remove();
clearInterval(balloonInterval);
// Check if game over
if (missed >= 20) {
alert(`Game Over! You popped ${score} balloons.`);
clearInterval(gameInterval);
}
}
}, Math.random() * 20 + 5);
}
// Game loop
let gameInterval = setInterval(createBalloon, 800);
restartButton.addEventListener('click', function() {
while (balloonContainer.firstChild) {
balloonContainer.removeChild(balloonContainer.firstChild);
}
score = 0;
missed = 0;
scoreDisplay.textContent = score;
missedDisplay.textContent = missed;
clearInterval(gameInterval);
gameInterval = setInterval(createBalloon, 800);
});
});
الأمر باللغة الإنجليزية لاستجابة أفضل
Create a ‘Balloon Pop’ game using HTML, CSS, and JavaScript. In this game, various-sized balloons float upwards at different speeds. Players must pop the balloons by clicking or tapping them to earn points. If 20 balloons escape without being clicked, the game ends and displays the total number of balloons popped. Include a ‘Restart Game’ button that allows players to reset everything and start fresh. Ensure the game is responsive on both desktop and mobile devices. Provide the complete code for the game, including the HTML layout, CSS styling, and interactive JavaScript functionalities.
Responses