برمجة لعبة مسابقات
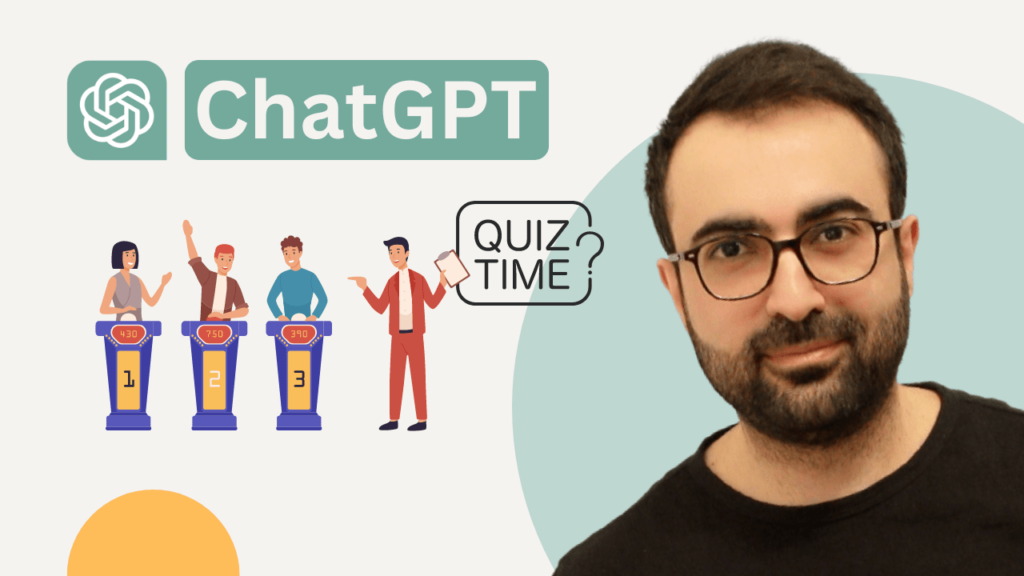
الأمر الموجه لـ ChatGPT
إنني أتطلع إلى تطوير لعبة اختبار بسيطة يمكن تشغيلها على متصفح الويب باستخدام HTML للتخطيط، وCSS للتصميم الأساسي، وJavaScript لآليات الاختبار. يجب أن تقدم اللعبة سلسلة من الأسئلة متعددة الاختيارات، واحداً تلو الآخر، بحيث يحتوي كل سؤال على أربع إجابات محتملة. يجب على المستخدمين تحديد إجابتهم من خلال النقر عليها. بمجرد إرسال الإجابة، يجب أن تشير اللعبة على الفور إلى ما إذا كانت الإجابة صحيحة أم خاطئة، وتحديث نتيجة المستخدم وفقاً لذلك، وعرض السؤال التالي. يجب أن يتم تتبع اللعبة وعرض النتيجة الحالية للمستخدم وتقدمه خلال الاختبار (على سبيل المثال، “السؤال 2 من 4”). أحتاج أيضاً إلى زر إعادة التشغيل لإعادة ضبط الاختبار في أي وقت. يجب أن يكون التصميم واضحاً وسريع الاستجابة ليناسب أحجام الشاشات المختلفة. هل يمكنك توفير الأكواد اللازمة لتنفيذ هذه الميزات؟
HTML (Structure)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Simple Quiz Game</title>
<link rel="stylesheet" href="styles.css">
</head>
<body>
<div class="quiz-container">
<div id="question" class="question"></div>
<div class="choices" id="choices"></div>
<button id="next-button" onclick="nextQuestion()">Next Question</button>
<button id="restart-button" onclick="restartQuiz()">Restart Quiz</button>
<div id="progress"></div>
<div id="score"></div>
</div>
<script src="script.js"></script>
</body>
</html>
CSS (Styling)
body {
font-family: Arial, sans-serif;
display: flex;
justify-content: center;
align-items: center;
height: 100vh;
margin: 0;
}
.quiz-container {
text-align: center;
width: 90%;
max-width: 600px;
background: #f4f4f4;
padding: 20px;
border-radius: 8px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.question {
font-size: 24px;
margin-bottom: 20px;
}
.choices {
margin: 10px 0;
}
.choices div {
background: #ddd;
padding: 10px 20px;
margin: 5px 0;
cursor: pointer;
border-radius: 5px;
}
.choices div:hover {
background: #ccc;
}
button {
padding: 10px 20px;
margin-top: 20px;
cursor: pointer;
background: blue;
color: white;
border: none;
border-radius: 5px;
}
button:hover {
background: darkblue;
}
#score {
font-size: 18px;
margin-top: 20px;
}
JavaScript (Functionality)
const quizQuestions = [
{ question: "What is the capital of France?", answers: ["New York", "Berlin", "Paris", "London"], correct: 2 },
{ question: "What is 2 + 2?", answers: ["3", "4", "5", "6"], correct: 1 },
// Add more questions as needed
];
let currentQuestionIndex = 0;
let score = 0;
function displayQuestion() {
const question = quizQuestions[currentQuestionIndex];
document.getElementById("question").textContent = question.question;
const choicesContainer = document.getElementById("choices");
choicesContainer.innerHTML = '';
question.answers.forEach((answer, index) => {
const choice = document.createElement("div");
choice.textContent = answer;
choice.onclick = () => selectAnswer(index);
choicesContainer.appendChild(choice);
});
document.getElementById("progress").textContent = `Question ${currentQuestionIndex + 1} of ${quizQuestions.length}`;
document.getElementById("score").textContent = `Score: ${score}`;
}
function selectAnswer(index) {
const isCorrect = index === quizQuestions[currentQuestionIndex].correct;
if (isCorrect) {
score += 1;
}
document.getElementById("score").textContent = `Score: ${score}`;
nextQuestion();
}
function nextQuestion() {
currentQuestionIndex++;
if (currentQuestionIndex < quizQuestions.length) {
displayQuestion();
} else {
showResults();
}
}
function restartQuiz() {
currentQuestionIndex = 0;
score = 0;
displayQuestion();
}
function showResults() {
const questionElement = document.getElementById("question");
questionElement.textContent = "Quiz Completed!";
const choicesContainer = document.getElementById("choices");
choicesContainer.innerHTML = '';
document.getElementById("progress").textContent = "You've completed the quiz!";
}
document.addEventListener("DOMContentLoaded", displayQuestion);
الأمر باللغة الإنجليزية لاستجابة أفضل
I am looking to develop a Simple Quiz Game that can be played on a web browser using HTML for the layout, CSS for basic styling, and JavaScript for the quiz mechanics. The game should present a series of multiple-choice questions, one at a time, with each question having four possible answers. Users should select their answer by clicking on it. Once the answer is submitted, the game should immediately indicate if the answer was right or wrong, update the user’s score accordingly, and display the next question. The game should track and display the user’s current score and their progress through the quiz (e.g., ‘Question 5 of 20’). I also need a restart button to reset the quiz at any time. The design should be straightforward but responsive to accommodate different screen sizes. Can you provide the JavaScript code needed to implement these features?
Responses